number of elements in an array:
print, N_ELEMENTS(array)
unix command in idl: dollar sign ($) before command
$pwd
$ls
list all variables currently being used:
help
doc_library, 'spherematch' -- man page for the function
which, 'spherematch' --- tells you where in the IDL path the function is.
when recompiling idlutils need to use evilmake
!pi is constant pi
plothist (makes histogram plot)
when looking at a structure you use the /str command:
help, mystructure, /str
reading a fits file using mrdfits:
filedata = mrdfits(file2read, 1)
Combine two structures of the same size:
struct3 =struct_combine(struct1,struct2)
Concatenate two structure of the same type:
struct3 = [struct1, struct2]
Plotting Histogram (from here):
PRO t_histogramIf you add maxmatch=1 to your spherematch it will avoid duplicates (should default to this)
data = [[-5, 4, 2, -8, 1], $
[ 3, 0, 5, -5, 1], $
[ 6, -7, 4, -4, -8], $
[-1, -5, -14, 2, 1]]
hist = HISTOGRAM(data)
bins = FINDGEN(N_ELEMENTS(hist)) + MIN(data)
PRINT, MIN(hist)
PRINT, bins
SPLOT, bins, hist, YRANGE = [MIN(hist)-1, MAX(hist)+1], PSYM = 10, XTITLE = 'Bin Number', YTITLE = 'Density per Bin'
END
total with higher precision: total(...., /double)
in for loops:
for j = 0L, rsize-1 do begin
the L makes j a long int.
;Show color table
colorName = PickColorName(startColorName)
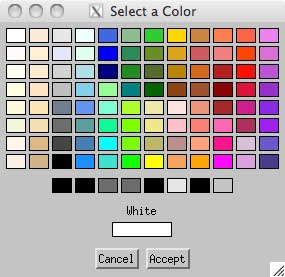
; Make heat map (warning, not very sophisticated)
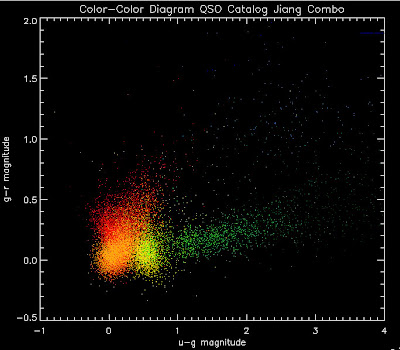
colorobject = qsotemplate1.z_sim
xobject = ugqcolor1
yobject = grqcolor1
colorbin = 0.3
colorstart = 1.0
colorstop = colorstart + colorbin
plot, xobject[0:1], yobject[0:1], ps=null, color=fsc_color('white'), xr=[-0.5,4],yr=[-0.5,2], XTITLE = 'u-g magnitude', YTITLE = 'g-r magnitude', TITLE = 'Color-Color Diagram QSO Catalog Jiang Combo', charsize = 1.5, charthick = 1
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('dark red') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('red') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('orange red') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('orange') ;Fake QSO
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('gold') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('lawn green') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('lime green') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('dark green') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('teal') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('dodger blue') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('royal blue') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('blue') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('navy') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('dark slate blue') ;Fake QSOs
colorstart = colorstop
colorstop = colorstart + colorbin
zrange = where(colorobject GE colorstart AND colorobject LT colorstop)
oplot, xobject[zrange], yobject[zrange], ps=3, color=FSC_COLOR('dark orchid') ;Fake QSOs
The hope is that I will expand on this list every time I come across something I think I might use again (but don't use enough to memorize).
Jessica,
ReplyDeleteFor Pi, use !DPi. It's always recommendable to use double precision with non-integers.